Protecting websites and mobile applications from bots and fraudulent users is a challenge. Captchas are no longer effective, and KYC processes can be costly. That’s why validating if users’ phone numbers are fake has become one of the most effective strategies against fraud.
Verifying users’ phone numbers with an OTP (One-Time Password) during registration has become one of the most effective methods to safeguard your website or application. Big platforms like Google, Facebook, or Instagram use this strategy to ensure the integrity and security of their user base. Indeed, requiring a valid phone number poses a significant challenge for bots due to the high cost of acquiring multiple numbers, unlike email addresses. For fraudsters, it’s also a hurdle since phone numbers are typically linked to real identities.
However, implement fake phone number validation is not a trivial task. It involves detecting incorrect formats, sending OTP codes, and blocking valid but untrustworthy numbers.
This article will explore various methods to implement fake phone number validation, including verifying the format, checking the line’s validity, and ensuring the number is not disposable. These steps will help you protect your website or application from bots and fraudsters.
Validate the Phone Number Format
Phone numbers can be presented in so many formats depending on the country, the purpose, and the context in which they are used.
The same number can be formatted in dozens of different ways. That makes it really difficult to validate a phone number in your application. In some countries, periods are used to separate numbers for better readability. Others use dashes, parentheses, or spaces.
Here are just a few examples:
- 2123456318
- 212-345-6318
- (212)345-6318
- (212)-345-6318
- 212.345.6318
- +12123456318
- 212 345 6318
- +212-345-6318
- 1-212-345-6318
- +1 212.345.6318
Always use the E.164 international format
Don’t even try to validate all these different formats: there are too many possible variations and too many formats to consider. Instead, use the international E.164 format, which standardizes everything.
E.164 establishes a universal format for international telephone numbers. With this standard, phone numbers are restricted to digits only and can be up to fifteen digits in length. The format includes a country code, which is one to three digits long, and a telephone number, which can be up to twelve digits long.
Country | E.164 Phone Number Example |
---|---|
United States 🇺🇸 | +12123456318 |
United Kingdom 🇬🇧 | +441234567890 |
France 🇫🇷 | +33123456789 |
Germany 🇩🇪 | +491234567890 |
Japan 🇯🇵 | +811234567890 |
In your registration form, we recommend integrating a JavaScript plugin that provides a versatile phone number input field. This will allow users to enter their phone numbers in various formats, which are then automatically converted to the E.164 format before being sent to your backend. This way, you only need to verify that the format complies with E.164 standards. You don't have to deal with other formats. It simplifies your phone number validation process!
JavaScript librairies for inputting telephone numbers
International Telephone Input
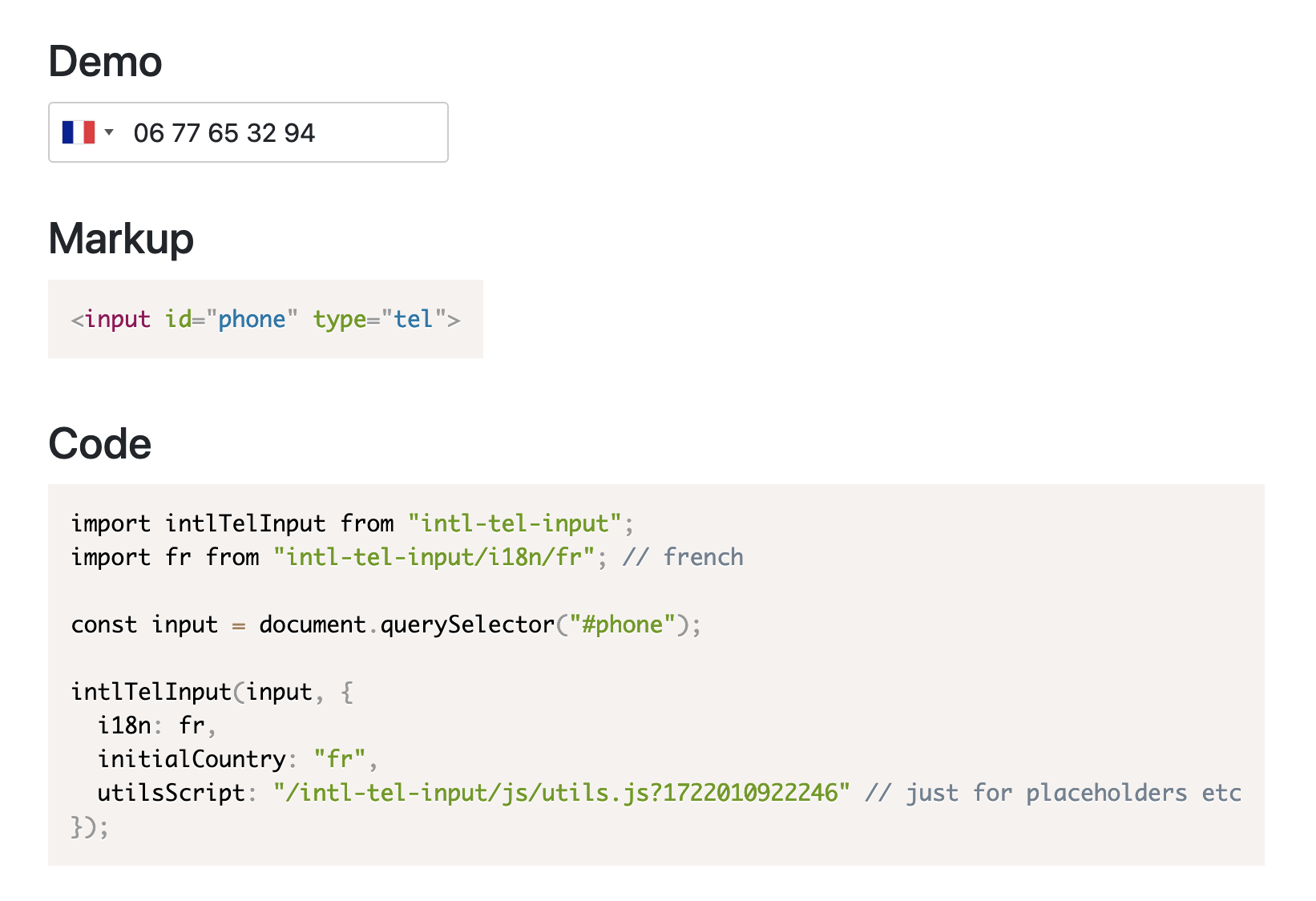
- GitHub repository
- Demo
- Vanilla JS support: Yes ✅
- React support: Yes ✅
- Vue JS support: No ❌
International Telephone Input is a JavaScript plugin designed for inputting and validating phone numbers. It transforms a standard input field by adding a searchable country dropdown, automatically detecting the user’s country, and formatting the number as it is typed.
React Phone Number Input
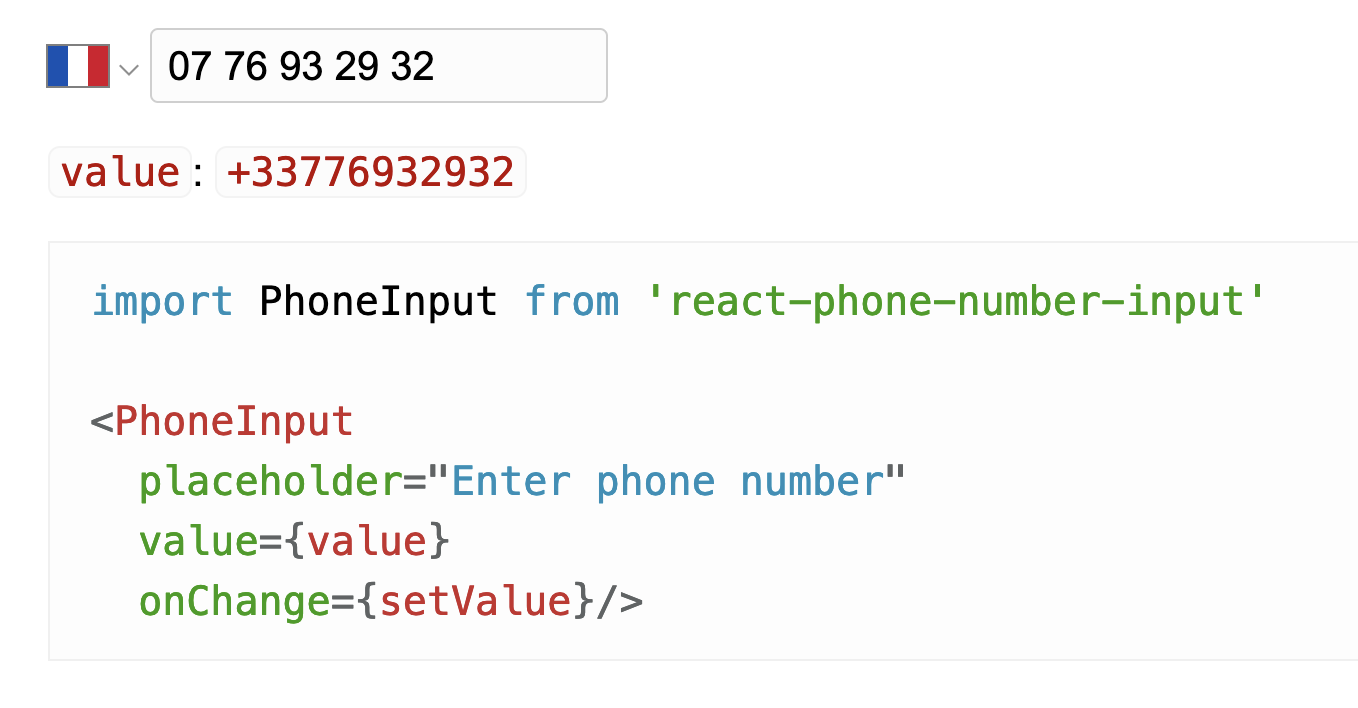
React component for international phone number input.
Regex to validate E.164 format
You’ve implemented some JavaScript to always receive E.164 formatted phone numbers on your back end? That’s great! But you still need to validate that the input you received matches E.164 rules.
How do you do that? In short, use a regular expression. You can do that in any programming language.
Here is a regex to validate E.164 formatted phone numbers:
^\+[1-9]\d{1,14}$
This regex matches strings that start with a +, followed by a digit between 1 and 9, and then followed by 1 to 14 more digits.
Validate E.164 phone numbers in PHP
function validatePhoneNumber($number) {
return preg_match('/^\+[1-9]\d{1,14}$/', $number);
}
validatePhoneNumber('+1234567890'); // true
validatePhoneNumber('123456'); // false
Validate E.164 phone numbers in Python
import re
def validate_phone_number(number):
pattern = r'^\+[1-9]\d{1,14}$'
return bool(re.match(pattern, number))
validate_phone_number('+1234567890') // True
validate_phone_number('123456') // False
Validate E.164 phone numbers in Ruby
def validate_phone_number(phone_number)
!!(phone_number =~ /^\+[1-9]\d{1,14}$/)
end
puts validate_phone_number('+1234567890') // true
puts validate_phone_number('123456') // false
Validate E.164 phone numbers in JavaScript
function validatePhoneNumber(number) {
const pattern = /^\+[1-9]\d{1,14}$/;
return pattern.test(phoneNumber);
}
validatePhoneNumber('+1234567890'); // true
validatePhoneNumber('123456'); // false
Verify that the Phone Number is Not Disposable
At this step, we have received a correctly formatted E.164 number. We still don't know if it's a real number or a fake one, but we are getting closer. We still need to verify the ownership of this number by sending a verification code (OTP) to the user. We’ll cover this process in detail in the next section.
However, before sending a SMS verification code, you must ensure that the phone number is not a disposable one. These type of numbers is called by different names:
- Disposable phone numbers
- Temporary phone numbers
- Free phone numbers
- Publicly shared phone numbers
All these terms refer to the same thing: active lines provided by certain websites solely for receiving SMS verification codes. These numbers cannot be used for making calls; they are often public numbers that forward received SMS messages to a public web page.
These websites typically rely on ads for revenue, which is why most of these numbers are free. It's ridiculously easy for bots and fraudsters to get one and create a fake account on your platform!
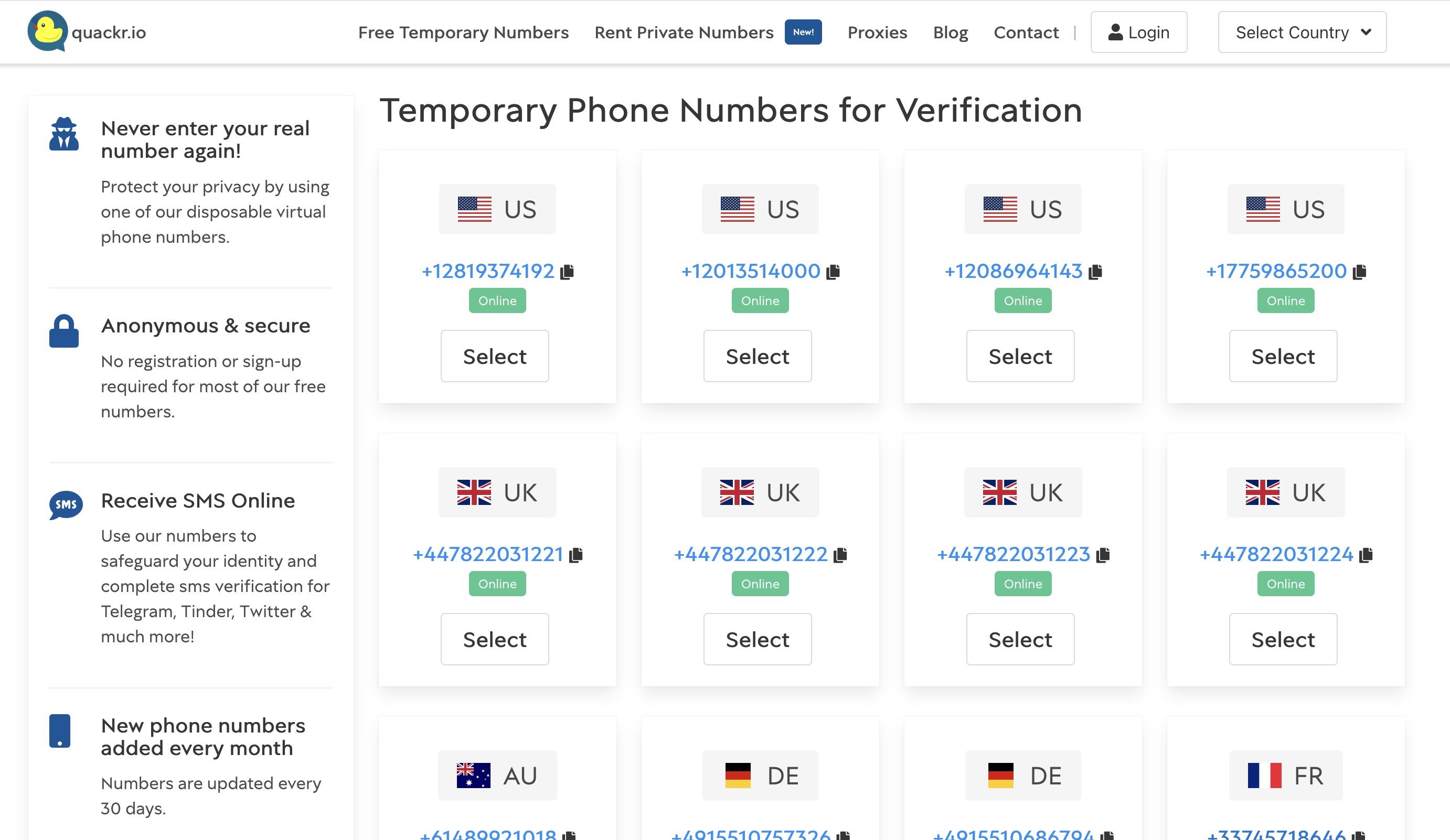
You absolutely need to avoid these disposable phone numbers. Bots use them to bypass verification systems, and fraudsters use them to hide their real numbers. If you don’t detect them, your OTP system is useless. But detecting them is a complex task: they change constantly, with hundreds of new numbers being created every day.
This is our area of expertise. We monitor the web in real time to identify these numbers the moment they are published and make them available to you through our API. You can use it to check whether a phone number is disposable or legitimate.
NumCheckr API
It can be up and running in less than 10 minutes:
- Create a NumCheckr account.
- Generate an API token on this page.
- Call our API to verify a phone number.
Here is an example of how to call our API to verify a phone number:
curl -X POST numcheckr.com/api/check-number \
-H "Authorization: Bearer <YOUR-API-TOKEN-SECRET-VALUE>" \
-H "Content-Type: application/json" \
-d '{ "phone": "+447497265710" }'
It returns a JSON response telling you whether the phone number is disposable or not.
{
"active_line": true,
"is_disposable": true,
"public_sms_gateways": [
"online-sms.org",
"sms24.me"
],
"type": "MOBILE",
"country_code": "gb",
"country_name": "United Kingdom",
"carrier": "EE",
"e164_format": "+447497265710",
"national_format": "07497 265710",
"international_format": "+44 7497 265710"
...
}
Verify the Line Ownership
We have verified that the number is correctly formatted and that it is not a disposable line. All that remains is to verify that the line actually belongs to the user registering on your platform.
To do this, the best option is to implement a OTP (one-time password) verification mechanism, which is relatively simple:
- Generate a random code of 4 to 6 digits.
- Store it in your database and associate it with the user ID.
- Send an SMS to the user.
- Ask the user to enter the code received by SMS to proceed with registration.
You can code this yourself or use libraries. It’s not very complicated; it’s just about generating, storing, and sending a random code. However, you might be wondering how to send an SMS to the user. At NumCheckr, we do not offer this service, but many services provide APIs for sending SMS.
Based on our experience, we can suggest a few options.
Which platform to choose for sending SMS OTP verification codes?
Twilio
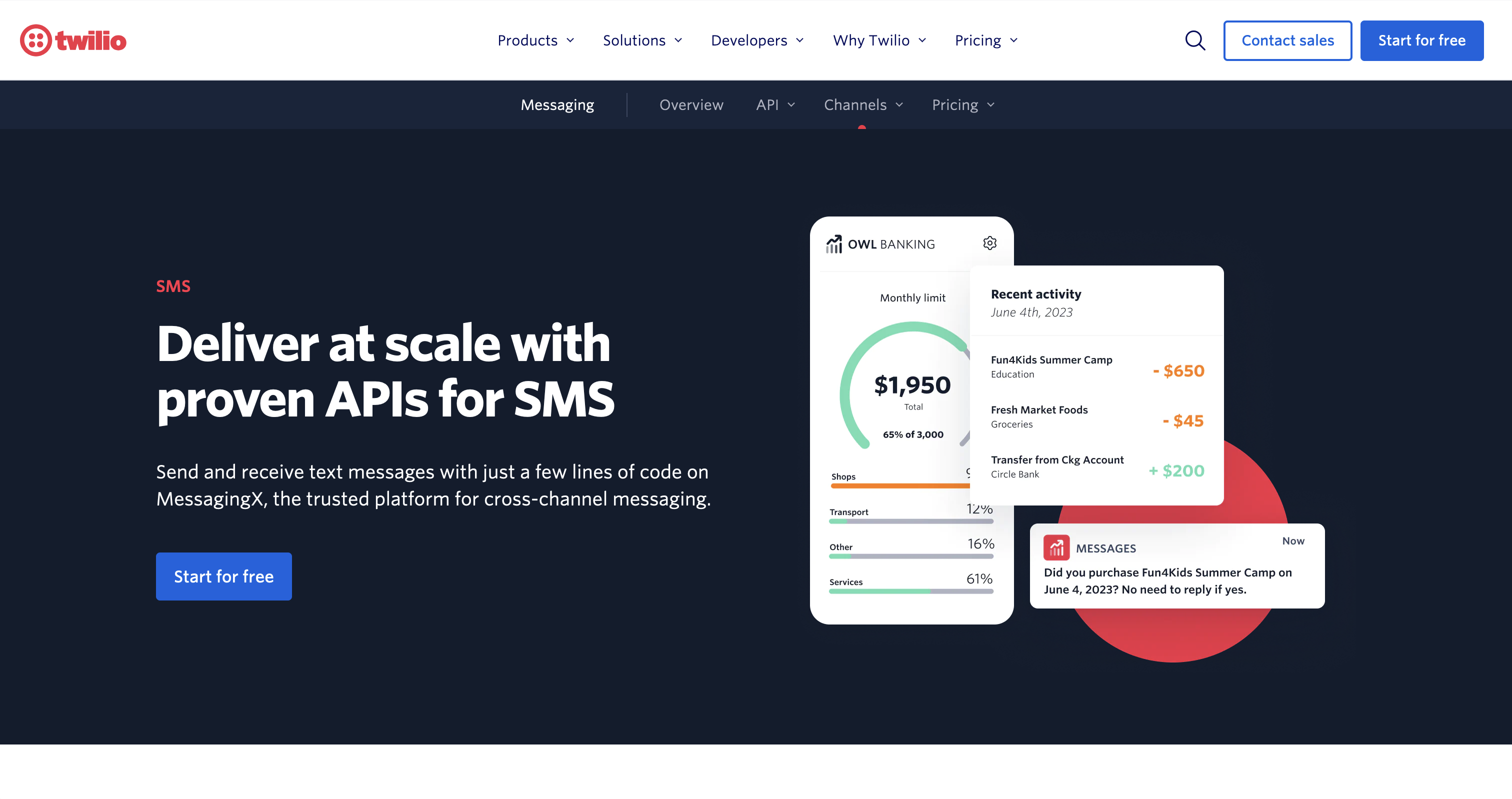
Founded in 2008, Twilio provides a simple API to send SMS messages across 180 countries. Their API is always up and their dashboard is nice. The cost is $0.0079 per SMS.
Twilio SMS pricingVonage
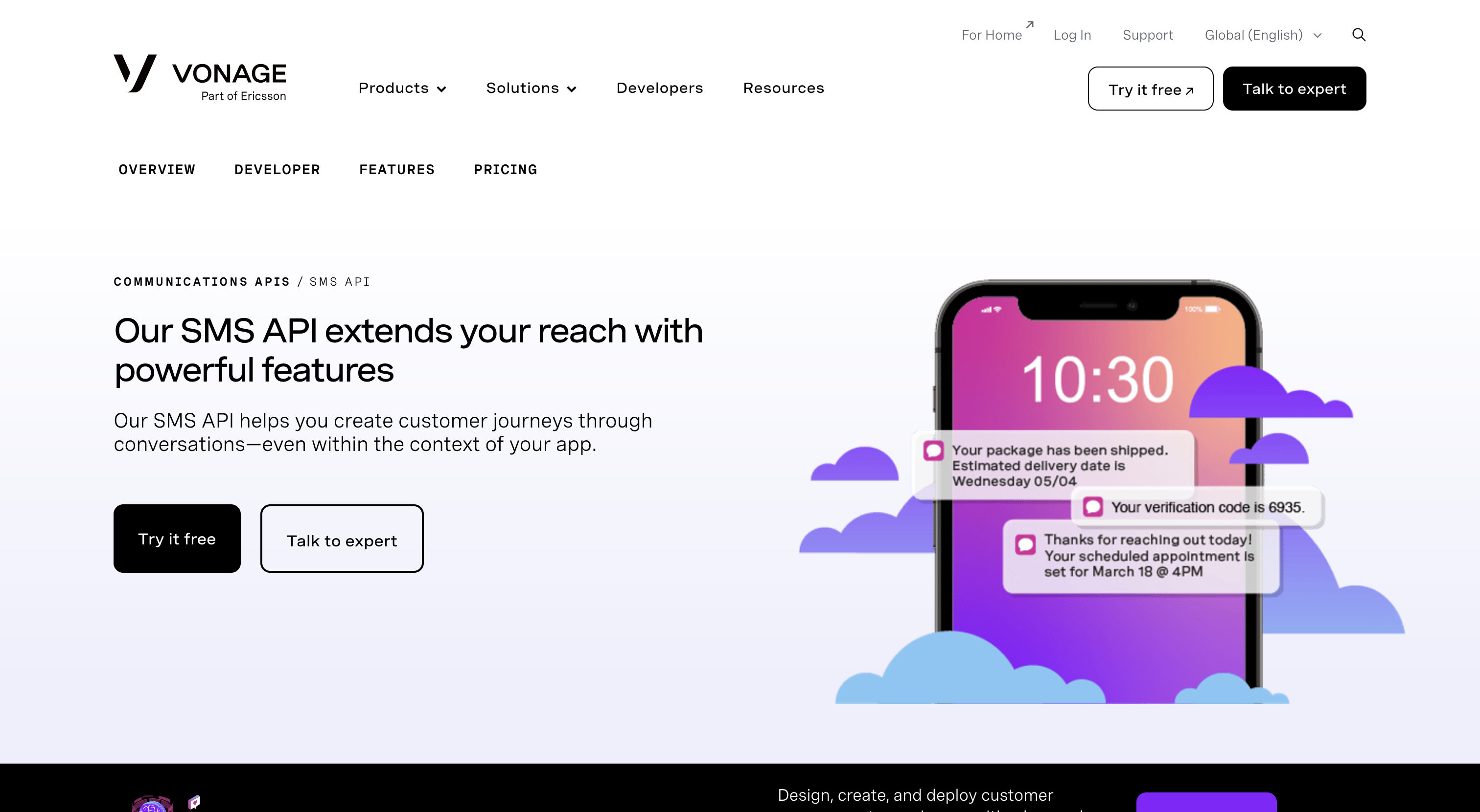
Previously known as Nexmo, also provides a straightforward API to send text messages. The cost is $0.0770 per SMS.
Vonage SMS pricingHonestly, both are great options. Just choose the one you prefer.
Conclusion
Verifying whether a phone number is fake or legitimate is the best way to combat bots, spammers, and fraudsters. However, it’s not that simple and it needs to be done at multiple levels:
- Verify the phone number format
- Check if the phone number is disposable or not
- Verify the ownership of the line
The second step, checking if the number is disposable, is crucial; without it, you will end up wasting money on OTP codes. To help with this, feel free to try our API (first lookups are free!) and implement it on your platform, in your new account verification process.
By following these steps, you’ll build a strong verification system that protects your application from fake numbers and malicious users.